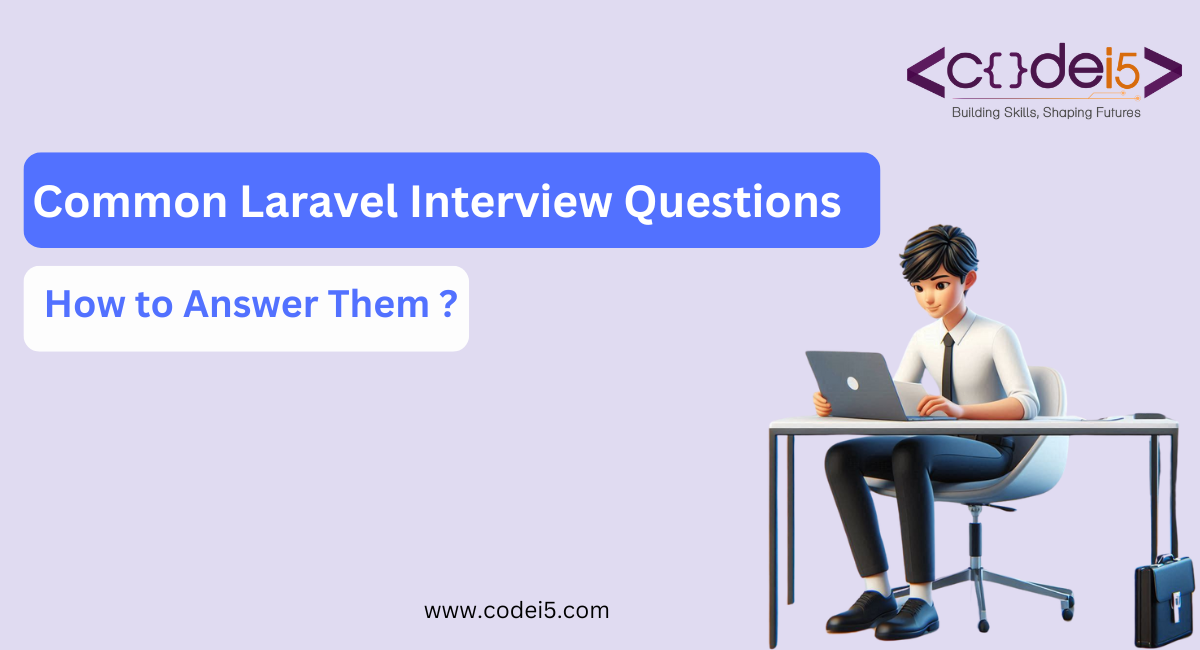
Introduction to Laravel
What is Laravel?
Laravel is a widely-used, open-source PHP framework designed to simplify the process of web application development. It follows the MVC (Model-View-Controller) architectural pattern, which helps organize code by separating business logic from the user interface. This structure makes it easier for developers to maintain and scale their applications.
Key Features of Laravel
- Eloquent ORM: A powerful Object-Relational Mapping tool that allows developers to interact with the database using a simple, expressive syntax instead of complex SQL queries.
- Routing: An intuitive routing system that enables developers to define URL routes effortlessly, linking them to specific controller actions.
- Blade Templating Engine: A flexible templating engine that facilitates the creation of dynamic views with features like template inheritance and sections.
- Artisan CLI: A command-line interface that provides helpful commands for tasks such as database migrations, seeding, and testing, streamlining the development process.
- Security: Built-in security features protect applications against common vulnerabilities like SQL injection, cross-site request forgery (CSRF), and cross-site scripting (XSS).
- Testing Support: Laravel offers robust support for unit and feature testing, allowing developers to ensure their applications function as expected.
- Task Scheduling: Simplifies the automation of repetitive tasks through a clean syntax, eliminating the need for manual cron job setups.
- Community and Documentation: With a large and active community, along with comprehensive documentation, developers can easily find support and resources.
Basic Concepts
What is MVC and How Does Laravel Implement It?
MVC (Model-View-Controller) is a design pattern that organizes an application into three components:
- Model: Manages data and business logic (handled by Eloquent in Laravel).
- View: Displays data to the user (created with the Blade templating engine).
- Controller: Handles user input and interacts with the Model and View.
Laravel implements MVC by clearly separating these components, making the code easier to maintain and develop.
Explain Routing in Laravel
Routing in Laravel defines the paths for your application. It maps URL patterns to specific controller actions.
- Defining Routes: Routes are set in the web.php file. For example:
Route::get('/home', [HomeController::class, 'index']);
- Route Parameters: You can create dynamic routes:
Route::get('/user/{id}', [UserController::class, 'show']);
This allows for clean and user-friendly URLs.
What are Middleware and How Do They Work?
Middleware in Laravel filters HTTP requests entering your application. It can perform tasks like authentication and logging.
- Functionality: Middleware checks requests before reaching routes, allowing you to enforce rules, like checking if a user is logged in.
- Creating Middleware: Use Artisan to create custom middleware:
php artisan make:middleware CheckAuth
- Applying Middleware: Attach it to routes easily:
Route::get('/dashboard', [DashboardController::class, 'index'])->middleware('checkAuth');
Middleware enhances request handling efficiently.
Database Interactions
How does Eloquent ORM work?
Eloquent ORM lets you interact with your database using simple PHP code. Each database table corresponds to a model, allowing you to perform actions like retrieving, inserting, and updating records without writing complex SQL. It also supports relationships between tables for easier data management.
What are Migrations and Seeders?
· Migrations: These are like version control for your database. They let you define and modify your database structure using PHP, making it easy to share changes with your team.
· Seeders: Seeders help you fill your database with sample or default data. They allow you to quickly populate tables with records for testing or initial setup.
Explain Query Builder vs. Eloquent
· Query Builder: A flexible way to build SQL queries using a fluent interface. It’s great for complex queries where you need more control.
· Eloquent: An object-oriented approach that makes it easy to interact with your tables as if they were classes. It’s more readable for simple operations
Database Interactions
How does Eloquent ORM work?
Eloquent ORM lets you interact with your database using simple PHP code. Each database table corresponds to a model, allowing you to perform actions like retrieving, inserting, and updating records without writing complex SQL. It also supports relationships between tables for easier data management.
What are Migrations and Seeders?
· Migrations: These are like version control for your database. They let you define and modify your database structure using PHP, making it easy to share changes with your team.
· Seeders: Seeders help you fill your database with sample or default data. They allow you to quickly populate tables with records for testing or initial setup.
Explain Query Builder vs. Eloquent
· Query Builder: A flexible way to build SQL queries using a fluent interface. It’s great for complex queries where you need more control.
· Eloquent: An object-oriented approach that makes it easy to interact with your tables as if they were classes. It’s more readable for simple operations
Authentication and Authorization
How Does Laravel Handle Authentication?
Laravel offers a built-in authentication system that makes it easy to manage user login and registration. It includes features for user registration, login, password resets, and email verification. The system uses the Auth facade to check if a user is logged in and to access user information. Laravel supports various authentication methods, such as session-based and API token authentication.
What is the Difference Between Authorization and Authentication in Laravel?
· Authentication: Verifies the identity of a user. It confirms whether the user is who they say they are, usually through credentials like a username and password.
· Authorization: Determines what an authenticated user can do. It checks the user’s permissions and roles to see if they can access certain resources or perform specific actions.
In short, authentication is about identity verification, while authorization is about permissions. These concepts are crucial for Laravel interview questions.
Testing in Laravel
How Do You Write Tests in Laravel?
In Laravel, you write tests using the built-in testing framework that extends PHPUnit. You can create tests for various parts of your application, such as unit tests, feature tests, and browser tests.
- Create a Test: Use Artisan commands to generate a new test file.
- Define Test Methods: Inside the test class, write methods that assert expected outcomes, using Laravel’s helpful testing helpers.
- Run Tests: Use the command line to execute your tests and see results.
Laravel makes it easy to test routes, database interactions, and more, ensuring your application functions as intended.
What is PHPUnit and Its Role in Laravel Testing?
PHPUnit is a widely-used testing framework for PHP that provides tools for writing and running tests. In Laravel, PHPUnit is the foundation of the testing system.
- Test Structure: Laravel’s tests extend PHPUnit’s features, allowing you to leverage its assertions and setup methods.
- Running Tests: PHPUnit handles the execution of tests and generates reports on their outcomes.
Overall, PHPUnit is essential for ensuring the reliability of Laravel applications, making it a key topic in Laravel interview questions.
Best Practices
To ensure clean and efficient Laravel development, follow these best practices: Use Eloquent ORM for database interactions and adhere to PSR standards for consistent coding. Implement route model binding to simplify routing and keep controllers slim by moving business logic to service classes. Utilize middleware for tasks like authentication and write unit and feature tests for reliability.
For project structure, follow the MVC pattern, use service providers for bootstrapping, and group routes by functionality. Store configuration in the config directory and organize Blade views into feature-based subdirectories. Finally, use .env files for environment-specific settings. These practices enhance the maintainability of Laravel applications, important for Laravel interview questions.
Conclusion
Adhering to best practices in Laravel development is essential for creating maintainable and scalable applications. By using Eloquent ORM, following PSR standards, and structuring your project effectively, you enhance code quality and workflow. Understanding key concepts like authentication, authorization, testing, and database interactions will prepare you for Laravel interview questions.
For interview success, focus on code quality and real-world applications. Consider utilizing resources like CodeI5 Academy, which offers training and insights to strengthen your skills and readiness for Laravel roles.