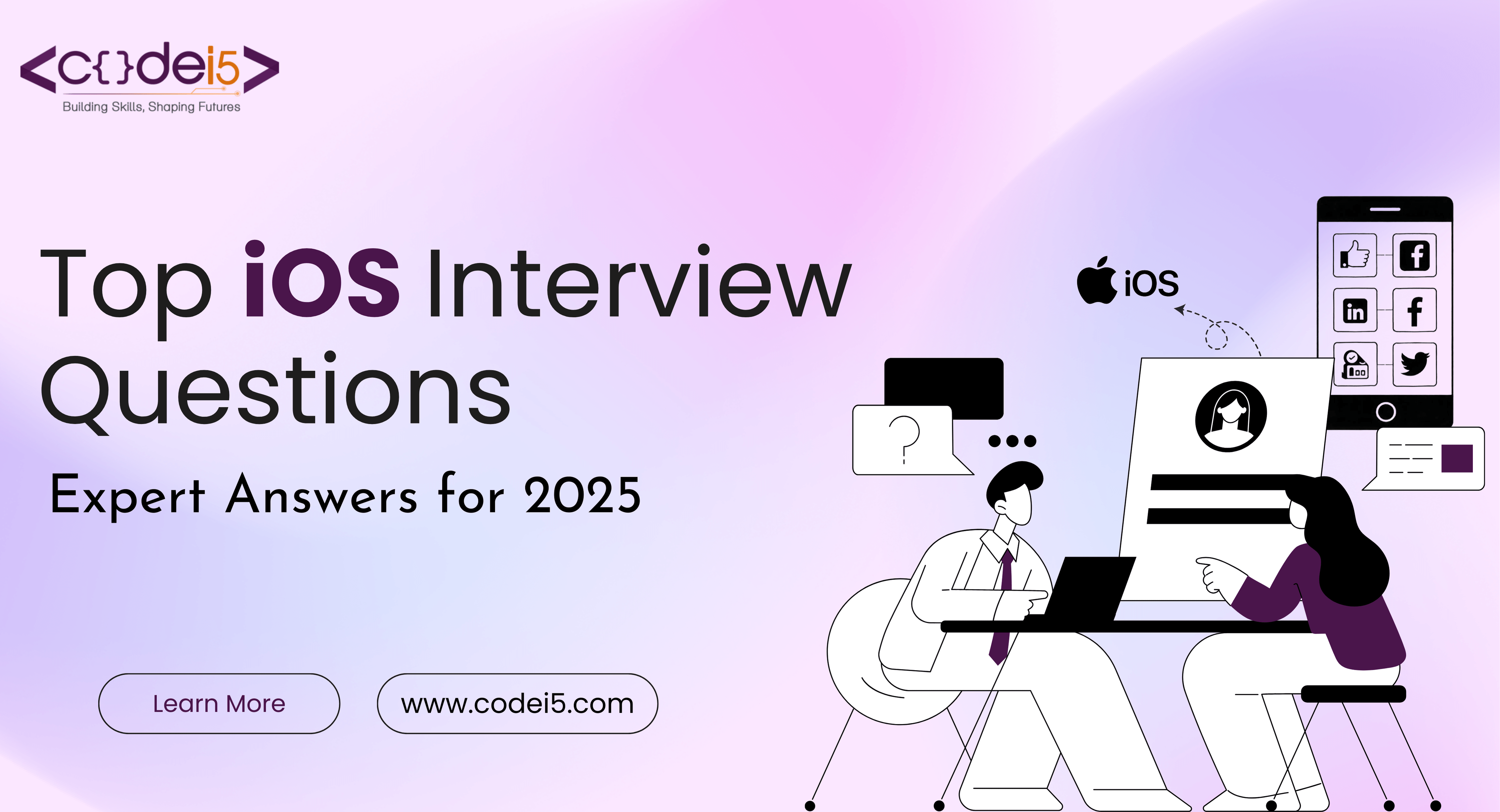
If you’re an iOS developer (or hoping to be one), you’ve probably heard it before: the interview process is tough. Whether you’re just starting out or you’ve been coding for a while, facing those tricky iOS questions can be a major challenge. It’s not just about knowing Swift—things like new tools, frameworks, and problem-solving are all on the table.
Every developer has faced those tough interview moments, and with the right prep, you can totally own it. In this blog, we’re going to break down the key iOS interview questions you’ll face in 2025. With clear, expert answers, you’ll be equipped to turn those challenges into wins and nail your next interview!
Why iOS Development Remains a Top Career Choice?
iOS development is a great career choice for many reasons:
1. High Demand and Good Salaries
- Strong Job Market: Apple’s devices are very popular, so there’s always a need for iOS developers.
- Good Pay: iOS developers earn competitive salaries because their skills are in high demand.
2. Modern Technology
- Swift Programming Language: Swift is easy to learn, fast, and safe, making it great for building apps.
- Powerful Tools: iOS developers use tools like SwiftUI, Core Data, and Core Animation to create great apps.
3. Easy-to-Use Development Environment
- Xcode: Xcode is a software that makes it easy to write, test, and fix iOS apps.
- Design Guidelines: Apple provides simple rules for making apps that are easy to use and look great.
4. App Store Opportunities
- Global Reach: The App Store allows developers to reach millions of users worldwide.
- Earning Potential: Developers can earn money from their apps through in-app purchases, subscriptions, and ads.
5. Always Learning and Growing
- New Technology: Apple regularly updates its tools and technology, keeping iOS development fresh and exciting.
- Stay Updated: Developers must keep learning to stay on top of the latest trends and best practices.
With these benefits, iOS development is a great career choice that offers job security, exciting challenges, and good pay.
How to Prepare for iOS Developer Interviews in 2025?
To ace your iOS developer interview in 2025, focus on these key areas:
1. Core Concepts
- Swift: Learn the basics (variables, functions) and advanced topics (generics, closures).
- SwiftUI: Understand declarative syntax, state management, and layouts (stacks, lists, grids).
- UIKit: Know views, view controllers, Auto Layout, and table/collection views.
2. iOS Frameworks
- Core Data: Learn data modeling, fetching, and saving data.
- Core Animation: Get familiar with basic and custom animations.
- Networking: Use URLSession for network requests and handle JSON data.
3. Design Patterns
- Learn MVC, MVVM, and VIPER patterns.
- Understand dependency injection for cleaner code.
4. Performance
- Use Instruments to optimize performance (memory, CPU, startup time).
- Learn background tasks for long-running operations.
5. Interview Tips
- Practice Coding: Use LeetCode and HackerRank for Swift coding challenges.
- Review Common Questions: Prepare answers for typical iOS interview questions.
- Build Projects: Show real-world apps you’ve created.
- Stay Updated: Follow Apple’s latest updates and documentation.
By focusing on these areas, you’ll be ready for your iOS developer interview. Good luck!
Let’s Dive Deeper into Each Area
Swift Programming Language
- Core Concepts:
- Classes vs Structures: Learn their differences, inheritance, and polymorphism.
- Protocols: Understand how to define and use them.
- Generics: Use type parameters and constraints.
- Closures: Learn how closures work and how to use them.
- Advanced Topics:
- Property Wrappers: Learn @State, @Binding, and @ObservedObject.
- Result Builders: Use for creating complex UI.
- Concurrency: Understand async/await and task groups.
- Best Practices:
- Optimize Code: Use lazy properties and early returns.
- Memory Management: Understand strong, weak, and unowned references.
- Error Handling: Use try-catch to manage errors.
UIKit and SwiftUI
- UI Design and Layout:
- Master Auto Layout and use Stack Views.
- Learn SwiftUI’s declarative syntax for views.
- User Interface Components:
- Customize Table and Collection Views.
- Create custom views with UIView or UIViewRepresentable.
- Manage state with @State, @Binding, and @ObservedObject.
- State Management:
- Use Combine for reactive programming.
- Understand SwiftUI’s unidirectional data flow.
iOS Frameworks and APIs
- Foundation Framework:
- Use Core Foundation for low-level tasks.
- Make network requests with URLSession.
- Core Data:
- Model and save data using NSManagedObjectContext.
- Optimize with NSFetchedResultsController.
- Core Graphics and Core Animation:
- Draw graphics with Core Graphics.
- Animate with Core Animation.
iOS Architecture Patterns
- MVC, MVVM, and VIPER:
- Learn when to use each design pattern.
- Dependency Injection:
- Manage dependencies using Swinject.
- Testing:
- Write unit and UI tests using XCTest.
iOS Performance Optimization
- Profiling Tools:
- Use Instruments to monitor performance.
- Memory Management:
- Use ARC and avoid memory leaks.
- App Startup Time:
- Speed up app launch by deferring tasks.
Top iOS Interview Questions to Prepare For:
Swift Questions
- What’s the difference between classes and structures in Swift?
- Classes: Reference types, support inheritance, and can have deinitializers.
- Structures: Value types, copied by value, and don’t support inheritance.
- When to Use: Use classes when inheritance, identity, or deinitialization is needed. Use structures when working with value types.
- What is a protocol in Swift?
- A protocol defines a set of methods, properties, and requirements that a class, struct, or enum must implement.
- Unlike classes, protocols only specify what a type should do, not how to do it.
- Example: A protocol can specify that a class must have a function
performAction()
but does not define how the action is performed.
- What is the purpose of generics in Swift?
- Generics enable you to write flexible and reusable code. You can create functions, methods, and types that work with any data type.
- Example: A generic function can work with Int, String, or any other type, making it reusable without rewriting the code for each type.
- What are property wrappers in Swift?
- Property wrappers allow you to add custom behavior to properties. Swift provides built-in property wrappers like
@State
,@Binding
, and@ObservedObject
for managing state in SwiftUI. - Use: Property wrappers are used to add additional functionality, like managing state or performing side effects, without modifying the core logic of your code.
- Property wrappers allow you to add custom behavior to properties. Swift provides built-in property wrappers like
- How does concurrency work in Swift?
- Swift uses async/await and actors for concurrency.
- Async/await allows writing asynchronous code in a sequential manner.
- Actors provide thread-safe storage and data handling in concurrent environments.
- Example:
async
allows you to perform tasks like network requests without blocking the UI.
UIKit & SwiftUI Questions
- What’s the difference between Auto Layout and Stack Views?
- Auto Layout: Offers flexibility in designing complex layouts by setting constraints for each element. It’s powerful but requires more setup.
- Stack Views: Simplifies layout by arranging views in vertical or horizontal stacks. Great for simpler layouts where automatic alignment and spacing are required.
- How do you create a custom view in SwiftUI?
- Use a struct that conforms to the View protocol.
- Define the
body
property that describes the content and layout of the view. - Apply modifiers to adjust appearance and behavior.
- Explain state and data flow in SwiftUI.
- @State: Manages mutable state within a view (e.g., tracking a button click).
- @Binding: Allows one view to share state with another.
- @ObservedObject: Observes changes in an external object and updates the view.
- Data Flow: Data flows in one direction—from parent to child views.
- How do you handle asynchronous operations in SwiftUI?
- Use Task to perform asynchronous operations like network requests or data loading.
- Use
Task.result.value
to handle success, andTask.result.error
for failure handling. - You can also use
async/await
for cleaner asynchronous code.
iOS Frameworks & APIs Questions
- What is Core Data and how is it used for data persistence?
- Core Data is an object graph and persistence framework. It is used to manage and persist data across app sessions.
- You define entities (data models) and their attributes (data fields).
- NSManagedObjectContext is used to save, fetch, and delete data.
- What is the difference between Core Graphics and Core Animation?
- Core Graphics: A low-level framework for drawing 2D graphics (e.g., lines, shapes, images).
- Core Animation: A high-level framework for creating animations and transitions for views and layers.
- How do you make network requests in iOS?
- Use URLSession to create and manage network requests.
- Common task types include URLSessionDataTask, URLSessionDownloadTask, and URLSessionUploadTask.
- Handle errors, timeouts, and asynchronous operations with completion handlers.
Architecture Patterns Questions
What are the advantages and disadvantages of MVVM?
- Advantages:
- Clear separation of concerns between UI and business logic.
- Easier to test, more maintainable code.
- Reusability of view models.
- Disadvantages:
- Can add complexity to smaller apps.
- Requires more boilerplate code than MVC.
- What is dependency injection, and how does it work?
- Dependency injection is a technique to provide the dependencies (objects or services) that a class or function needs.
- It helps improve modularity and testability.
- Common techniques: Property Injection, Method Injection, and using libraries like Swinject.
- What are best practices for writing unit tests?
- Write small, focused tests.
- Mock objects should be used to isolate components.
- Ensure each test only tests one piece of functionality.
- Use a testing framework like XCTest to run and manage tests.
Performance Optimization Questions
- How do you measure app performance?
- Use Instruments to profile app performance (CPU usage, memory usage, and frame rate).
- Look for memory leaks, slow network requests, or inefficient algorithms that might affect performance.
- What are common performance bottlenecks in iOS apps?
- Slow network requests or high latency.
- Inefficient algorithms that take too long to execute.
- Memory leaks, leading to increased memory usage.
- Long UI rendering times causing the app to freeze or slow down.
- How can you optimize app startup time?
- Defer non-critical tasks (e.g., loading data, initializing services) to background threads.
- Optimize the app launch sequence by reducing the number of initializers.
- Use lazy loading for resources that aren’t needed immediately.
Conclusion
To do well in your iOS developer interview in 2025, focus on mastering Swift, SwiftUI, and key iOS frameworks. Understand the basics, explore advanced topics like property wrappers and concurrency, and practice code optimization and memory management.
Learn how to design UI using Auto Layout, Stack Views, and SwiftUI, and understand state management. Be familiar with Core Data, Core Graphics, and Core Animation.
Use good architecture patterns like MVC, MVVM, and VIPER. Write unit and UI tests, and improve your app’s performance with profiling tools.
Keep practicing, stay updated, and communicate your ideas clearly. You’ll be ready for success in your iOS interview!
If you’re looking to build a strong foundation, consider joining Codei5 Academy’s iOS Developer course in Coimbatore, available both as live and online classes. You’ll be ready for success in your iOS interview!