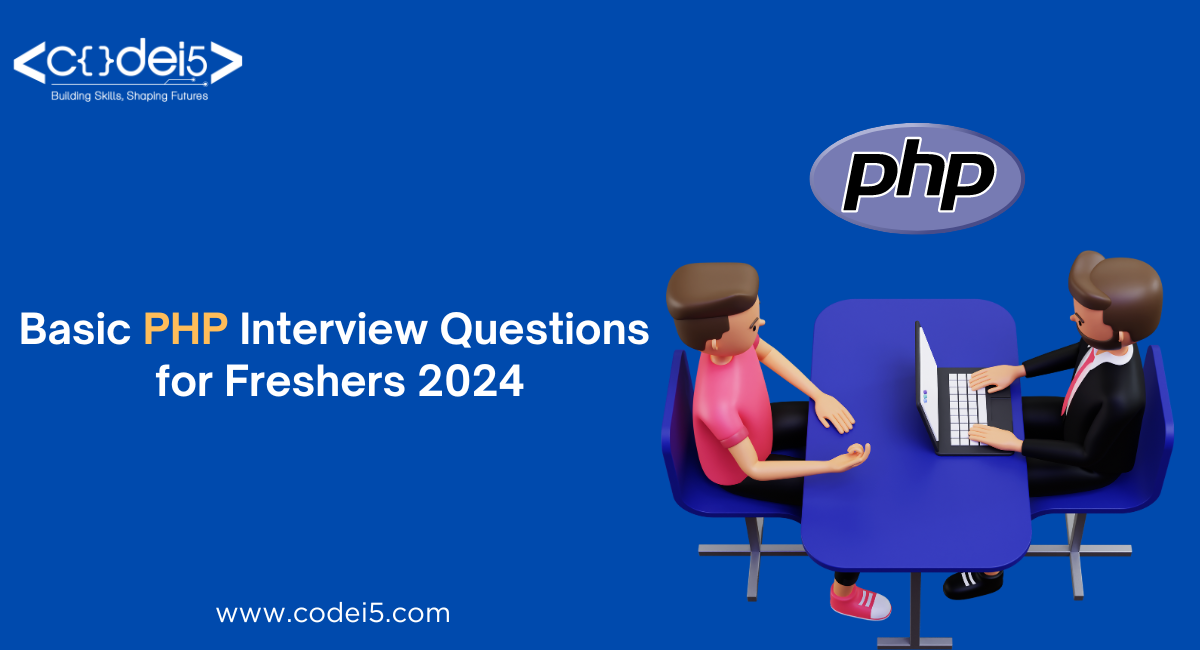
You’ve spent countless hours wrestling with syntax, debugging your code until 3 AM, and dreaming in arrays. You’ve conquered the world of echo and print, and now you’re ready to step into the lion’s den: the PHP interview.
We’re here to make the PHP interview process simple and clear. We’ll break down tricky ideas into easy bits. From the basics to the more detailed topics, we’ve got you covered. So, grab a cup of coffee, get comfortable, and let’s start this coding journey together!
Think you know PHP? Think again. This isn’t just a blog; it’s your tactical guide to turning PHP knowledge into interview gold. We’re talking about the kind of knowledge that makes interviewers do a double-take, followed by a job offer.
Are you ready to transform from a PHP novice to a confident interview pro? Ready to go from a PHP coder to a PHP ninja? Let’s sharpen those coding skills and prepare to reveal your inner interview champion!
Let’s get started with these essential PHP interview questions!
What is PHP?
PHP stands for “Hypertext Preprocessor.” It is a server-side scripting language designed for web development. In simple terms, PHP is used to create dynamic and interactive websites. Unlike HTML, which is static and only shows what you put on the page, PHP can change the content of a page based on user actions or data.
A Brief History of PHP
PHP was originally created by Rasmus Lerdorf in 1994 as a simple set of Common Gateway Interface (CGI) scripts written in C. Over the years, it has evolved into a powerful and feature-rich language. PHP 3, released in 1998, marked a significant milestone with improved object-oriented capabilities. Today, PHP is in its 8th major version, offering enhanced performance, security, and new language constructs.
Basic Syntax and Structure
PHP code is typically embedded within HTML files using special tags: <?php and ?>. Here’s a simple example:
PHP
<?php
echo “Hello, world!”;
?>
Explanation:
- PHP Tags (<?php and ?>):
- <?php starts the PHP code block.
- ?> ends the PHP code block.
- The server processes everything between these tags as PHP code.
- echo Statement:
- echo outputs text to the web page.
- echo “Hello, world!”; sends the text “Hello, world!” to the browser.
Result:
When the server runs this PHP code, it sends “Hello, world!” to the browser. The user sees this message on their web page.
Data Types in PHP
PHP is a dynamically typed language, which means you don’t need to specify the type of a variable when you create it. Here are the main data types you’ll work with in PHP:
- Integers: Whole numbers, like 10 or -5.
- Floating-point numbers: Numbers with decimal points, like 3.14 or 2.5.
- Strings: Text sequences, like “Hello”.
- Booleans: Values that can be true or false.
- Arrays: Lists of values, such as a list of names or numbers.
- Null: Represents no value at all.
Variables, Constants, and Operators
- Variables: Store data that can change. They start with a dollar sign ($). For example, $name might hold a person’s name.
- Constants: Store values that do not change throughout the script. They are defined once and remain the same throughout. For example, PI could be a constant representing the value of π (pi).
- Operators: Perform operations on variables and values. Common types include:
Arithmetic Operators: For basic math operations like addition and subtraction.
Comparison Operators: For comparing values, such as checking if one value is equal to another.
Logical Operators: For combining or inverting boolean values, like checking if multiple conditions are true.
Control Flow Statements
Control flow statements help decide which code runs and when. Common ones include:
- if-else: Decides which block of code to execute based on a condition.
- switch: Chooses which block of code to execute based on a variable’s value.
- Loops: Repeat code multiple times. Examples include while, for, and do-while loops.
Arrays
Arrays are used to store multiple values in one variable. PHP supports two main types:
- Indexed Arrays: Use numeric keys to access values, like using a number to refer to an item in the list.
- Associative Arrays: Use named keys to access values, like using a name to refer to an item in the list.
This overview provides a foundation for understanding PHP data types, variables, constants, operators, control flow statements, and arrays, which are essential for writing effective PHP code.
PHP and Databases
Introduction to Databases
A database is like a digital filing cabinet that organizes and stores data. It’s divided into tables, which are similar to spreadsheets with rows and columns. For example, a “users” table might have columns for name, email, and password, with each row representing a different user.
Connecting to Databases Using PHP
To work with a database from PHP, you need to connect to it, like opening the filing cabinet. There are two main ways to connect:
- MySQLi: Designed for MySQL databases.
- PDO (PHP Data Objects): Works with many types of databases.
SQL Queries and PHP
SQL (Structured Query Language) is used to communicate with and manage databases. PHP allows you to run SQL commands and handle the results.
For example, to get all users from the “users” table, you use the SQL command:
SELECT * FROM users;
Database Operations (CRUD)
CRUD stands for Create, Read, Update, and Delete. These are the basic actions you can perform:
- Create: Add new data (e.g., a new user).
- Read: Retrieve existing data (e.g., a list of users).
- Update: Change existing data (e.g., update a user’s email).
- Delete: Remove data (e.g., delete a user).
These basics will help you understand how to manage and use databases with PHP.
Common PHP Interview Questions
A Curated List of Frequently Asked PHP Interview Questions
PHP is a popular language with many potential interview questions. Here’s a list of common topics you might encounter:
Fundamental PHP
- What is PHP, and how is it different from other scripting languages?
- What are the different data types in PHP?
- What are variables and constants in PHP?
- What are control flow statements in PHP, and how do they work?
- How do you handle errors and exceptions in PHP?
- What is the difference between include and require?
Object-Oriented PHP
- What are the main concepts of Object-Oriented Programming (OOP) in PHP, like inheritance, polymorphism, and encapsulation?
- What are abstract classes and interfaces?
- How do you use namespaces in PHP?
- What are traits, and how do they differ from inheritance?
PHP and Databases
- How can you connect to databases in PHP? (e.g., MySQLi, PDO)
- How do you perform CRUD operations in PHP?
- What are prepared statements, and why are they important?
- How do you optimize database queries?
PHP and Web Development
- What is the difference between GET and POST methods?
- How do sessions and cookies work in PHP?
- What are $_SESSION and $_COOKIE superglobals used for?
- How do you handle file uploads in PHP?
- What is the MVC (Model-View-Controller) architecture?
Advanced PHP Topics
- What is the difference between early and late static binding?
- What is dependency injection?
- How do you handle security in PHP applications (e.g., SQL injection, XSS, CSRF)?
- What are some common design patterns used in PHP?
- How do you improve PHP performance?
Sample Answers and Explanations
Good answers usually include:
- A clear explanation of the concept.
- Practical examples or code snippets.
- Understanding the concept’s impact.
For example, to explain the difference between GET and POST methods:
“GET and POST are methods used to send data to a server. GET adds data to the URL, while POST sends data in the request body. GET is often used to retrieve data, while POST is used for submitting forms or creating data.”
Tips for Answering PHP Interview Questions Effectively
- Understand the question: Make sure you know what is being asked before you start answering.
- Structure your answer: Organize your thoughts and provide a clear response.
- Be specific: Use examples and details to support your answer.
- Highlight your skills: Talk about your relevant experience and projects.
- Ask clarifying questions: If you don’t understand something, ask for more details.
- Practice: Rehearse common questions and time your answers.
- Show enthusiasm: Demonstrate your interest in PHP and web development.
By preparing well and following these tips, you’ll be ready to impress in your PHP interview.
Conclusion
We’ve covered a lot in this guide, from the basics of PHP to how it works with databases. By understanding these key concepts, you’ve built a strong base for learning PHP. Remember, PHP is a flexible language with many possibilities.
There’s always more to learn beyond this guide. Exploring new PHP features, libraries, and frameworks will help you stay updated in web development.
If you’re feeling a bit lost or want a clear learning path, think about joining a PHP course. A good course can speed up your learning and help you prepare for real-world problems. Codei5 Academy offers a great PHP course that can give you the skills you need to succeed in PHP development and ace job interviews.
Keep practicing, keep learning, and keep experimenting! The world of PHP is vast and exciting – enjoy the journey!