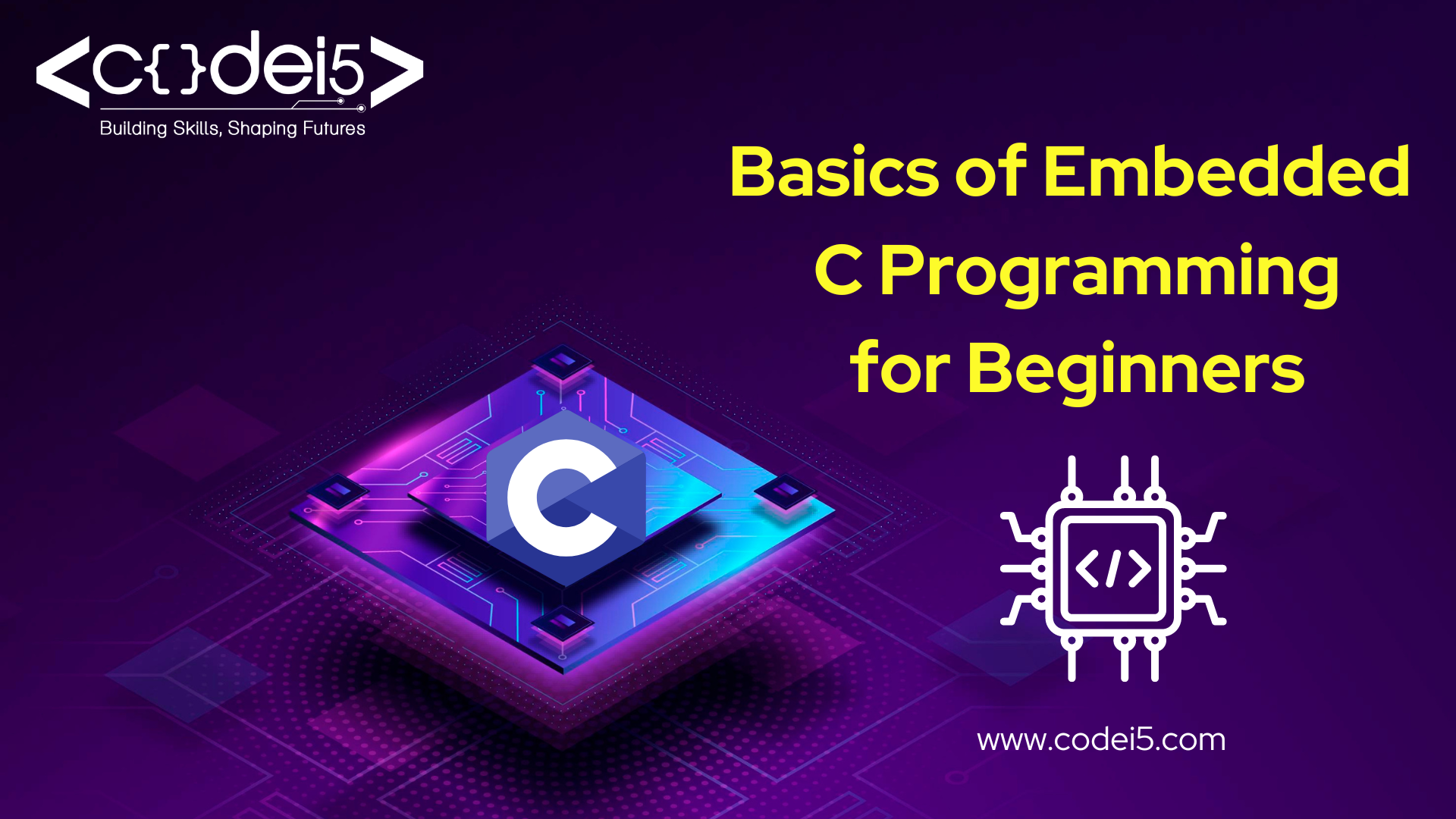
Do you find the small computers inside devices like smartphones and traffic lights fascinating? Do you dream of creating your own smart systems? If so, learning Embedded C programming is the first step towards making these dreams a reality!
What is Embedded C Programming?
Embedded C is a extension of the C programming language specifically designed for Embedded Systems. These are small, dedicated computers that are integrated within larger devices to control their functionality. From washing machines to robots, countless devices rely on embedded systems for their operation.
Why should you learn Embedded C?
Here are a few good reasons:
- High Demand: Companies in many industries need developers who know Embedded C, so it’s a skill that can lead to a stable and rewarding career.
- Versatility: Embedded C is used in a lot of different things, so once you learn it, you can work on all sorts of projects.
- Challenge and Reward: Getting really good at Embedded C means you’ll understand how hardware and software work together, which can be both challenging and satisfying.
The Foundation of Embedded C
Before we start coding, let’s cover some basic concepts:
Microcontrollers (MCUs): These are tiny computers embedded in devices. They contain a CPU, memory, and I/O peripherals to interact with the outside world.
Integrated Development Environment (IDE): This software helps you write, compile, debug, and download code onto the microcontroller. Beginner-friendly IDEs include Keil MDK, Arduino IDE, and Eclipse with CDT plugin.
Compilers: These tools convert your human-readable C code into machine code that the microcontroller can understand.
Building Blocks of Embedded C
- Data Types: These define the type and size of data your program can handle, like int (integer), float (floating-point number), and char (character).
- Variables: Named storage locations that hold data, declared with a data type and name, and optionally an initial value.
- Operators: Perform operations on data, like arithmetic (+, -, *, /), logical (&&, ||, !), and comparison (==, !=, <, >).
- Control Flow Statements: Control the program’s flow, including if-else, switch, for, and while loops.
- Functions: Reusable code blocks that perform specific tasks, taking input (arguments) and returning output (a value or action).
Embedded C Specifics
Embedded C adds hardware interaction features:
- Bit Manipulation: Deals with individual bits (0s and 1s) for hardware control, using operators like & (bitwise AND), | (bitwise OR), and << (left shift).
- Input/Output (I/O) Programming: Reading data from sensors (inputs) and controlling devices like LEDs (outputs) using specific microcontroller registers and functions.
- Interrupt Service Routines (ISRs): Special functions that respond to external events like button presses or timer overflows, allowing the program to react efficiently.
Beyond the Basics
As you advance, you’ll explore:
- Memory Management: Learn to allocate and manage memory efficiently, a critical skill in embedded systems where resources are limited.
- Real-Time Programming: Embedded systems often operate in real-time, requiring them to respond to events within strict time limits. Understanding real-time concepts is crucial.
- Hardware Interfacing: Explore communication protocols like I2C, SPI, and UART to connect the microcontroller with external devices such as sensors and displays.
Building Your First Embedded C Program: A Step-by-Step Guide
Now that you’ve understood the basics, let’s create a simple program! We’ll blink an LED connected to an Arduino Uno, a popular board for beginners.
What You’ll Need:
- Arduino Uno board
- Connecting wires
- LED
- Computer with Arduino IDE installed (download here)
Steps:
1. Connect the LED:
- Connect the longer leg of the LED to a 5V pin on the Arduino using a wire.
- Connect the shorter leg of the LED to a resistor (around 220 ohms), and then connect the other end of the resistor to a ground pin (GND) on the Arduino using another wire.
2. Open the Arduino IDE:
- Launch the Arduino IDE on your computer.
3. Create a new sketch:
- Go to File > New to create a new sketch.
4. Write the code:
#define ledpin 13
void setup() {
// initialize digital pin LED_BUILTIN as an output.
pinMode(ledpin, OUTPUT);
}
// the loop function runs over and over again forever
void loop() {
digitalWrite(ledpin,HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a second
digitalWrite(ledpin, LOW); // turn the LED off by making the voltage LOW
delay(1000); // wait for a second
}
5. Compile and upload:
- Click the “Verify” button to check for errors.
- If no errors, click the “Upload” button to upload the code to your Arduino.
Explanation:
- We define ledPin to store the pin number (13) connected to the LED.
- In setup(), we use pinMode(ledPin, OUTPUT) to set the pin as an output for the LED.
- In loop(), we use digitalWrite() to turn the LED on and off, with delay(1000) creating a 1-second interval.
Congratulations on creating your first embedded C program! As you progress, you can explore reading data from sensors, controlling motors, and interfacing with displays. The possibilities are endless!
Conclusion
Well done on completing this guide to embedded C programming! You now have a solid foundation for exploring this exciting field further. Remember, learning is a continuous journey, so don’t be afraid to experiment with projects and seek help from the community. With dedication and a bit of innovation, you’ll be well on your way to building amazing embedded systems with embedded C!