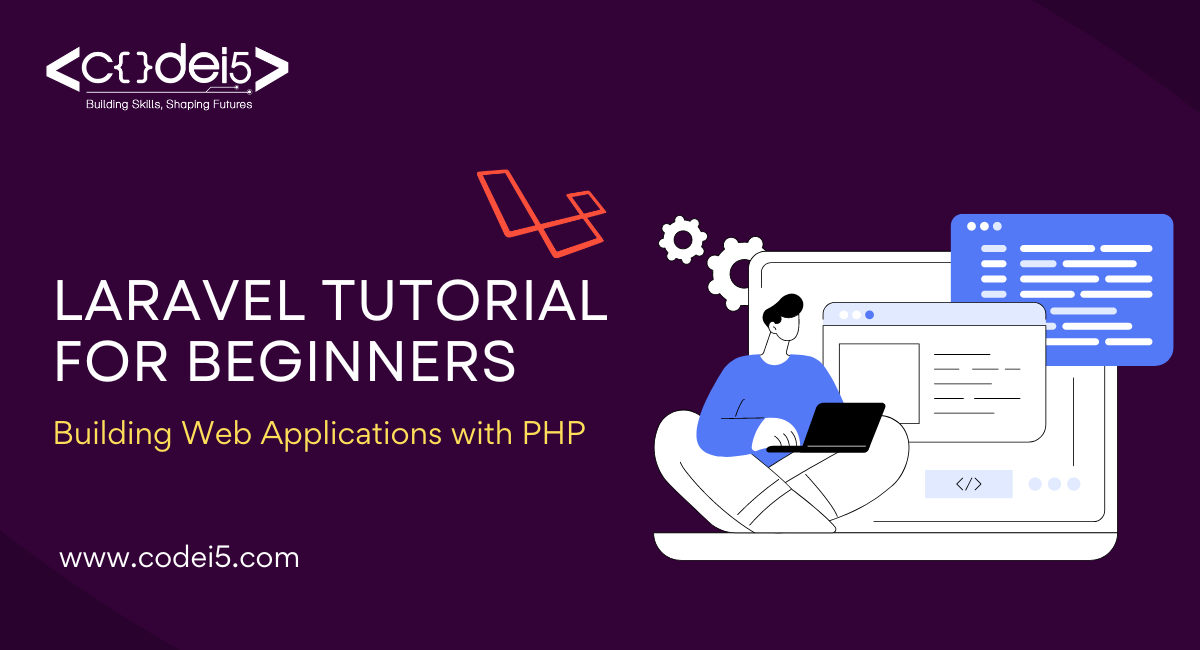
Laravel is a popular PHP framework that makes building web applications easier. In this tutorial, you’ll learn the basics of Laravel, from setting up your development environment to creating advanced applications. Whether you’re new to coding or have some experience, Laravel’s easy-to-understand syntax and powerful tools will help you build great web projects.
Laravel Overview
What is Laravel?
Laravel is a widely used, open-source PHP framework that makes it easier to develop web applications. It comes with a strong set of tools and features that help developers create modern, scalable, and maintainable applications.
Why Use Laravel?
- Simple Syntax: Laravel’s code is clean and easy to understand, making it beginner-friendly.
- Powerful Features: It includes useful tools like Eloquent ORM for databases, routing for managing URLs, templating with Blade, and built-in user authentication.
- Large Community: There’s a big, active community around Laravel that offers support, resources, and ready-to-use packages.
- Scalability: Laravel is built to handle high traffic and complex applications smoothly.
- Security: It follows best practices to keep your applications secure.
Advantages
- Fast Development: Laravel’s built-in features help you build applications quickly.
- Easy to Maintain: Laravel encourages writing clean and organized code, making it easier to maintain and update.
- Vast Ecosystem: Laravel has a rich ecosystem of packages and tools that extend its functionality.
- Community Support: Extensive documentation, tutorials, and forums are available, thanks to Laravel’s active community.
- Focus on Security: Laravel comes with security features built-in to protect your applications.
Key Features
- Eloquent ORM: A powerful tool for working with databases using PHP objects.
- Routing: A flexible system for managing how your application responds to URLs.
- Blade Templating: A simple engine for creating views with dynamic content.
- Authentication and Authorization: Ready-to-use features for managing users and permissions.
- Artisan CLI: A command-line tool for automating tasks and generating code.
- Queueing: A system for handling long-running tasks without slowing down your application.
- Caching: Tools to speed up your application by storing data for quick access.
- Testing: Built-in tools for testing your application’s code.
- Dependency Injection: A way to manage how different parts of your application interact.
- Modular Architecture: A flexible structure that makes your code easier to organize and manage.
Laravel – Installation
Prerequisites
Before you start installing Laravel, make sure you have the following:
- PHP: You need PHP version 8.1 or later. To check your PHP version, open your terminal and type php -v.
- Composer: Composer is a tool for managing PHP packages. You can download and install it from the official website: https://getcomposer.org/download/
- Web Server: Apache or Nginx is commonly used.
- Database: Laravel works with MySQL, PostgreSQL, SQL Server, or SQLite.
Installation Steps
1. Open a Terminal: Start by opening a terminal or command prompt on your computer.
2. Create a New Project Directory:
- Navigate to the folder where you want to create your Laravel project.
- Create a new directory for your project by running these commands:
mkdir my-laravel-project
cd my-laravel-project
3. Install Laravel Using Composer:
Run the following command to install Laravel:
composer create-project --prefer-dist laravel/laravel my-laravel-project
This will create a new Laravel project named my-laravel-project
in your current directory.
4. Start the Development Server:
After the installation is complete, start the development server by running this command in your project directory:
php artisan serve
The server will start, and you can access your Laravel application by going to http://127.0.0.1:8000 in your web browser.
Additional Notes
- Project Name: You can change
my-laravel-project
to any name you prefer for your project. - Installation Options: Besides Composer, you can also install Laravel using the Laravel Installer or Homestead. Check the official Laravel documentation for more details.
- Dependencies: Laravel automatically installs all the necessary packages and dependencies using Composer.
- Configuration: Laravel comes with configuration files that let you set up things like database connections and environment variables.
By following these steps, you’ll have a Laravel project ready to start developing!
Project Structure
The default Laravel project structure is well-organized, making it easier to keep your code clean and maintainable. Here’s a quick overview of the key directories:
- app: This is where the core logic of your application lives, including controllers, models, and services.
- config: Stores configuration files for different parts of your application, such as database connections, email settings, and more.
- database: Contains all your database-related files, including migration files (which define your database schema), seeders (for inserting test data), and factories (for generating fake data).
- public: The public-facing part of your application. It contains the
index.php
file (the main entry point), along with assets like JavaScript, CSS, and images. - resources: This directory holds your application’s resources, such as views (which are HTML templates), JavaScript files, and Sass files for styling.
- routes: Here you define how URLs in your application map to specific controller actions. It’s where you set up your application’s routing.
- storage: Stores files generated by your application, such as logs, uploaded files, and cached data.
- vendor: Contains all third-party libraries and dependencies managed by Composer.
Basic Configuration
1. Database Configuration:
Create a Database:
First, create a new database in your preferred database management system, like MySQL or PostgreSQL.
Update Configuration:
Next, you’ll need to tell Laravel how to connect to your database. Open the config/database.php
file and find the section for MySQL. Update it with your database details:
'mysql' => [
'driver' => 'mysql',
'host' => env('DB_HOST', '127.0.0.1'),
'port' => env('DB_PORT', '3306'),
'database' => env('DB_DATABASE', 'database_name'),
'username' => env('DB_USERNAME', 'your_username'),
'password' => env('DB_PASSWORD', 'your_password'),
'charset' => 'utf8mb4',
'collation' => 'utf8mb4_unicode_ci',
'prefix' => '',
'strict' => true,
'engine' => null,
],
Replace 'database_name'
, 'your_username'
, and 'your_password'
with your actual database name, username, and password.
2. Environment Configuration:
Laravel uses a file called .env
to store environment variables, which are settings your application needs to run. This file is in your project’s root directory.
Here’s an example of what it might look like:
APP_NAME=My Laravel App
APP_ENV=local
APP_DEBUG=true
APP_URL=http://localhost:8000
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=database_name
DB_USERNAME=your_username
DB_PASSWORD=your_password
Make sure to replace the placeholders with your actual information.
3. Additional Configuration:
You can configure other parts of your application, like mail settings, caching, and logging, by editing the files in the config
directory. For example, to set up email, you can update the config/mail.php
file with your email server details.
Note: When you deploy your application to a live server, it’s a good practice to store environment variables outside your codebase. This helps keep sensitive information, like database passwords, secure.
By following these simple steps, you’ll have a well-configured Laravel application ready for further development!
Core Concepts of Laravel
Laravel is a popular tool for building web applications using PHP. It comes with a lot of features that make development easier and more organized. Here’s a breakdown of its main ideas:
- MVC Architecture:
- Model: Handles the data and the logic behind it.
- View: Displays the data to the user (what the user sees).
- Controller: Connects the Model and View, managing the flow of data and responses.
- Eloquent ORM:
- Object-Relational Mapping: Connects your database tables to PHP classes, making it easier to work with data.
- Query Builder: A simple way to create and run database queries without writing complex SQL.
- Relationships: Lets you define how different pieces of data are connected, like how one user can have many posts.
- Routing:
- URL Mapping: Decides what happens when a user goes to a specific web address.
- Route Groups: Organizes similar routes together for better management.
- Named Routes: Gives names to routes, so you can easily refer to them later.
- Blade Templating Engine:
- Simple Syntax: Makes writing HTML mixed with PHP easier and cleaner.
- Directives: Provides special commands for tasks like loops and conditions.
- Sections: Helps you reuse parts of your code and customize layouts easily.
- Dependency Injection:
- Automatic Resolution: Automatically provides the necessary parts of your code where needed.
- Service Container: Manages the creation and lifecycle of objects in your app.
- Bindings: Defines how the different parts of your code are connected and provided.
- Artisan CLI:
- Command-Line Interface: A tool to run commands that help you manage your Laravel app, like creating new files or running tasks.
- Custom Commands: Allows you to create your own commands to do specific jobs.
- Middleware:
- Request/Response Handling: Controls what happens to requests before they reach your code and what happens to responses before they go back to the user.
- Authentication: Checks if users are logged in and allowed to do certain things.
- Rate Limiting: Limits how many times a user can make requests in a short period, to prevent overloads.
- Session Management:
- State Persistence: Remembers data across different user interactions.
- Flash Data: Temporarily stores data that’s only needed for a short time, like a success message after submitting a form.
- Queueing:
- Asynchronous Tasks: Runs long tasks in the background so the user doesn’t have to wait.
- Drivers: Supports different systems to handle these background tasks, like Redis or databases.
- Testing:
- Unit Testing: Tests small parts of your app to make sure they work correctly.
- Feature Testing: Tests how different parts of your app work together.
- Integration Testing: Tests how your app interacts with outside systems.
These concepts help developers build strong, easy-to-manage web applications with Laravel.
Building a Basic Laravel Application
CRUD Application
- Create Models, Migrations, and Seeders:
- Models: Define the data structure and rules.
- Migrations: Handle changes to your database setup.
- Seeders: Add initial data to your database.
- Define Routes:
- Set up paths that link to different parts of your application.
- Implement Controllers:
- Write the logic for actions like saving, updating, or deleting data.
- Create Views:
- Design the web pages users will interact with.
Form Handling
- Validation:
- Check user inputs to make sure they are correct.
- Redirects and Flash Messages:
- Send users to different pages after actions and show them temporary messages.
Session Management
- Storing Data:
- Save information temporarily for use across different pages.
- Retrieving Data:
- Access saved information when needed.
- Flash Messages:
- Display messages that last only for the next page load.
Advanced Topics
Authentication and Authorization
- User Registration and Login:
- Set up systems for users to sign up and log in.
- Password Hashing:
- Store passwords securely.
- Authentication Guards:
- Choose how to handle user authentication.
Middleware
- Creating Middleware:
- Build custom filters for requests.
- Applying Middleware:
- Attach filters to specific routes to control access.
Package Development
- Creating Packages:
- Organize and define the components of your own packages.
- Publishing Resources:
- Make your package’s configuration files, views, and assets available.
- Testing Packages:
- Write tests to ensure your package works correctly.
Testing
- Unit Tests:
- Test individual parts of your code.
- Integration Tests:
- Test how different parts of your application work together.
- Testing Tools:
- Use tools like PHPUnit to write and run tests.
Deployment
- Deployment Methods:
- Choose how to deploy your application, such as manually, via FTP, or with tools like Laravel Forge.
- Configuration:
- Set up your server environment, including database and settings.
Tips and Tricks
- Use Laravel’s Features: Take advantage of tools like Eloquent ORM and Blade templating.
- Read the Documentation: Check Laravel’s official documentation for guidance.
- Practice Often: Regular practice will help you improve.
- Join the Community: Engage with forums and communities for support and learning.
Conclusion
Laravel is a powerful tool for building web applications. If you’re new to Laravel and want to learn more, Codei5 Academy offers some of the best PHP courses to get you started.