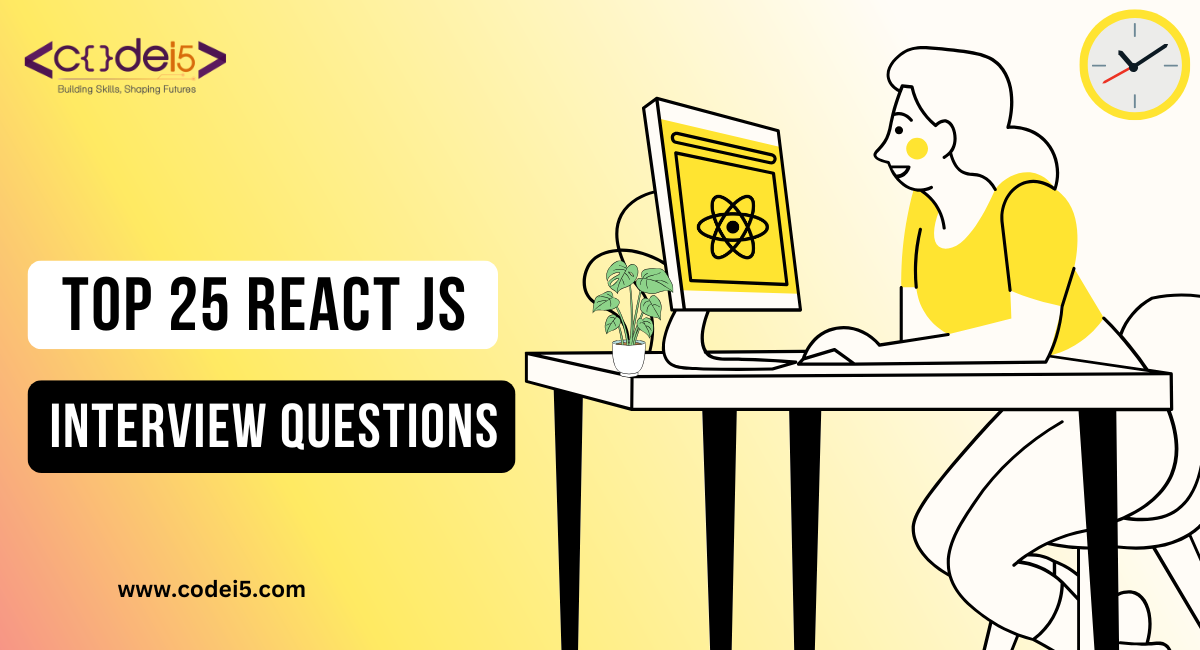
React JS is a popular JavaScript library created by Facebook that has completely changed how we build websites and apps. It’s loved by developers because it’s fast, easy to use, and helps create interactive user interfaces.
As we enter 2025, the demand for React developers is increasing. Many companies are using React for their web applications, so preparing well for a React JS interview is more important than ever.
In this blog, we’ll go through the top 25 React JS interview questions you need to know. These questions cover the basics, advanced topics, and real-world scenarios, giving you the knowledge to feel confident and perform well in your interview. Let’s get started!
React JS Basics: A Quick Recap
What is React JS?
React JS is a JavaScript library used to build user interfaces for websites and apps. It helps developers break down complicated designs into smaller, reusable pieces called components. This makes the code easier to organize, update, and reuse.
Key Features of React JS:
- Component-Based Architecture: Lets you create reusable pieces of UI, making development faster and more efficient.
- Virtual DOM: Speeds up updates by making changes in a lightweight copy (virtual DOM) before applying them to the real DOM.
- Unidirectional Data Flow: Keeps data flow predictable and easy to manage.
- JSX: A special syntax that looks like HTML but works with JavaScript to make UI creation simpler.
Why Understanding Basics is Important for Interviews?
Having a clear understanding of React JS basics is key to performing well in interviews. Employers often test how well you know these concepts:
- Lifecycle Methods: Know how to manage components at different stages (e.g., when they load, update, or get removed).
- Props and State: Understand the difference between props (unchangeable data) and state (changeable data) and how they work together.
- JSX: Be comfortable using JSX to create neat and readable UI components.
- Event Handling: Learn how to handle user actions like button clicks, typing, and form submissions.
By mastering these basic concepts, you’ll be ready to answer common React JS interview questions and prove your skills in creating reliable and efficient web applications.
Fundamental Concepts
1. What is React JS and How is It Different from Traditional Web Development?
React JS is a JavaScript library for building user interfaces. Here’s how it’s different:
- Component-Based Architecture: React divides the UI into reusable pieces called components, making the code easier to manage and update.
- Virtual DOM: Instead of updating the actual DOM directly, React uses a virtual DOM to track changes and update only the necessary parts, making it faster.
- Declarative Programming: In React, you describe how the UI should look, and React handles the rest, unlike traditional methods where developers manually update the DOM.
2. What is JSX? Why is It Used in React?
JSX (JavaScript XML) is a special syntax that lets you write HTML-like code inside JavaScript. It simplifies creating UI components by making the code easier to read and write.
How it works: React converts JSX into plain JavaScript that the browser understands.
Why use JSX?
- It makes UI code more readable and organized.
- It helps combine HTML and JavaScript logic in one place.
3. Class Components vs. Functional Components
Feature | Class Components | Functional Components |
Syntax | Use class keyword and extend React.Component . | Use simple JavaScript functions. |
State | Can manage state directly. | Use hooks (like useState ) to manage state. |
Lifecycle Methods | Have lifecycle methods like componentDidMount . | Use hooks (like useEffect ) for lifecycle tasks. |
Complexity | More complex and verbose. | Simpler and easier to write. |
4. What Are React’s Lifecycle Methods?
React components go through three main phases:
- Mounting (when the component is created)
constructor
: Sets up initial state.render
: Displays the component.componentDidMount
: Runs after the component is added to the DOM. Useful for fetching data or setting up listeners.
- Updating (when the component changes)
shouldComponentUpdate
: Decides if a component should re-render.render
: Updates the component’s UI.componentDidUpdate
: Runs after the update. Useful for tasks like updating the DOM or making API calls.
- Unmounting (when the component is removed)
componentWillUnmount
: Cleans up tasks like removing listeners or stopping timers.
5. What is the Virtual DOM and Why is It Important?
The virtual DOM is a lightweight copy of the actual DOM. When something changes in your app:
- React updates the virtual DOM first.
- It compares the new virtual DOM with the old one.
- React finds the smallest changes and updates only those parts in the actual DOM.
This process makes React apps faster because it avoids unnecessary updates to the real DOM, which is slower to manipulate.
6. What Are State and Props? How Are They Different?
Feature | State | Props |
Definition | Data managed inside a component. | Data passed from a parent component. |
Changeability | Can change (mutable). | Can’t change (immutable). |
Use Case | For data that a component manages itself. | For data a parent sends to a child. |
Example:
- State: Tracking whether a button is clicked inside the same component.
- Props: Sending a title or message from a parent to a child component.
Advanced Concepts
7. What is the Difference Between Shallow Rendering and Full Rendering in React Testing?
- Shallow Rendering: Tests only the component itself without rendering its child components. It’s helpful to check how the component behaves in isolation.
- Full Rendering: Renders the component along with its child components and simulates a real DOM. This is useful for testing interactions and state changes involving child components.
8. How Do You Optimize Performance in Large React Applications?
- Memoization: Use
React.memo
oruseMemo
to avoid redoing expensive calculations or re-rendering unchanged components. - Virtualization: Display only the visible parts of long lists or tables using libraries like React Virtualized.
- Code Splitting: Break your app into smaller bundles so it loads faster. Tools like React.lazy can help.
- Profiling: Use the React DevTools Profiler to find and fix slow parts of your app.
- Minimizing State Updates: Update only the parts of the state that are necessary to avoid unnecessary re-renders.
9. What Are Higher-Order Components (HOCs)?
HOCs are functions that take a component and return a new one with extra features.
Use Cases:
- Code Reuse: Share logic between multiple components.
- Conditional Rendering: Change what gets displayed based on conditions.
- Prop Manipulation: Add or modify props before passing them to a component.
10. What Are React Hooks and How Are They Different From Class Components?
React hooks are functions that let you use React features like state and lifecycle methods in functional components.
Difference:
- Hooks are simpler and work in functional components.
- Class components use lifecycle methods and require more code.
11. What is the useReducer Hook, and When Should You Use It?
useReducer
is a hook for managing more complex state logic.
When to Use:
- When the state has multiple parts or is complex.
- When state updates depend on the type of action (e.g., add, remove, update).
- When the logic involves calculations or multiple updates.
12. What Are useMemo and useCallback?
- useMemo: Caches the result of an expensive calculation and reuses it until the inputs change.
- Example: Avoid recalculating a filtered list every render.
- useCallback: Caches a function so it isn’t recreated every time the component re-renders.
- Example: Useful when passing functions as props to child components.
13. How Do You Handle Asynchronous Operations in React?
Use the useEffect
hook to manage side effects like fetching data.
Steps:
- Inside
useEffect
, usefetch
oraxios
to make an API call. - Handle the promise with
.then()
orasync/await
. - Update the component’s state with the fetched data.
14. What is Context API and How Does It Help?
The Context API allows you to share data between components without passing props through every level.
How It Works:
- Create a context object.
- Wrap components with a context provider to make data available.
- Use a context consumer or
useContext
hook to access the data in child components.
15. What Are Custom Hooks and Why Are They Useful?
Custom hooks are reusable functions that contain logic for managing state or side effects.
Benefits:
- Promote code reuse by sharing logic across components.
- Make code cleaner and easier to understand.
- Simplify testing by separating logic into smaller, testable functions.
Example: A custom hook for fetching data can be used in multiple components without rewriting the fetching logic.
React Router
16. What is React Router and How Does It Work?
React Router is a library that helps you manage navigation in a React app. It lets you define routes and show different components based on the URL in the browser.
17. How Do You Define Routes and Switch Between Them in React Router?
- Set Up the Router: Wrap your app with the
BrowserRouter
component to enable routing. - Define Routes: Use the
Route
component to set paths and link them to specific components. - Switch Between Routes: Use the
Switch
component to ensure only the first matching route is rendered.
Example:
import { BrowserRouter, Route, Switch } from 'react-router-dom';
function App() {
return (
<BrowserRouter>
<Switch>
<Route path="/" exact component={HomePage} />
<Route path="/about" component={AboutPage} />
</Switch>
</BrowserRouter>
);
}
18. What Are Nested Routes and Dynamic Routing?
1. Nested Routes:
- Define routes inside other routes to create a hierarchy.Useful for apps with sections or subsections.
Example:
<Route path="/dashboard">
<Dashboard>
<Route path="/dashboard/settings" component={Settings} />
</Dashboard>
</Route>
2. Dynamic Routing:
- Use parameters in the route (e.g.,
/profile/:id
) to handle different paths dynamically. - The parameter (e.g.,
id
) can be accessed inside the component.
19. How Do You Handle Navigation and URL Parameters in React Router?
1. Navigation:
- Use the
useNavigate
hook (oruseHistory
in older versions) to move to different routes programmatically.
const navigate = useNavigate();
navigate("/home");
2. URL Parameters:
- Use the
useParams
hook to access parameters from the URL.
import { useParams } from 'react-router-dom';
function Profile() {
const { id } = useParams();
return <div>Profile ID: {id}</div>;
}
This makes it easy to navigate between pages and work with dynamic data in your app.
React Testing
20. What Are Jest and React Testing Library?
- Jest: A testing framework for JavaScript. It helps write and run tests and comes with useful features like mocking and timers.
- React Testing Library: A tool for testing React components by simulating user interactions. It focuses on testing how components behave and what users see on the screen.
21. How to Write Unit Tests for React Components?
Unit tests check if a component works as expected. With React Testing Library, you can:
- Render the Component: Use the
render
function to load your component in the test. - Simulate User Actions: Use helpers like
fireEvent
oruserEvent
to simulate clicks, typing, etc. - Check the Output: Use assertions to verify the component behaves as expected (e.g., checks text, buttons, or DOM changes).
Example:
import { render, fireEvent } from '@testing-library/react';
import MyComponent from './MyComponent';
test('should display text when button is clicked', () => {
const { getByText } = render(<MyComponent />);
const button = getByText('Click Me');
fireEvent.click(button);
expect(getByText('Hello, World!')).toBeInTheDocument();
});
22. What is Snapshot Testing?
- Snapshot Testing: Captures a component’s UI as a snapshot (a saved file).
- How It Works: When you run the test again, it compares the current UI with the snapshot to check for unexpected changes.
- Benefits:
- Quickly finds visual bugs.
- Ensures UI consistency.
Example:
import { render } from '@testing-library/react';
import MyComponent from './MyComponent';
test('matches snapshot', () => {
const { asFragment } = render(<MyComponent />);
expect(asFragment()).toMatchSnapshot();
});
23. How to Test Asynchronous Operations?
When testing components that fetch data or perform async actions:
- Use the
act
function to wait for the operation to finish. - Check the component’s state or DOM after the async operation completes.
Example:
import { render, screen, waitFor } from '@testing-library/react';
import MyComponent from './MyComponent';
test('displays data after fetch', async () => {
render(<MyComponent />);
await waitFor(() => expect(screen.getByText('Data Loaded')).toBeInTheDocument());
});
React and Redux
24. What is Redux and How Does It Work with React?
Redux is a tool that helps manage state (data) for your entire app. It works by:
- State: All app data is stored in one place (the store).
- Actions: Messages (objects) that describe what needs to change in the state.
- Reducers: Functions that take the current state and an action, then return the new state.
In React:
- Use the
Provider
component to make the Redux store available to the app. - Use
useSelector
to read data from the store. - Use
useDispatch
to send actions to the store.
25. Core Concepts of Redux
1. State:
- Centralized data for the app.
- Example:
const initialState = { count: 0 };
2. Actions:
- Objects that describe what you want to do.
- Example:
const incrementAction = { type: 'INCREMENT' };
3. Reducers:
- Functions that decide how the state changes based on the action.
- Example:
function reducer(state, action) {
if (action.type === 'INCREMENT') {
return { ...state, count: state.count + 1 };
}
return state;
}
By understanding these concepts, you can manage even complex app data with ease!
Pro Tips for Cracking React JS Interviews
Here are simple and effective tips to help you succeed in your next React JS interview:
Technical Preparation
- Master the Basics:
- Component Lifecycle Methods: Learn how and when to use these methods.
- JSX: Understand the syntax and why it makes React development easier.
- Virtual DOM: Know how it improves performance by efficiently updating the UI.
- State vs. Props: Understand their differences and when to use each.
- Hooks: Get comfortable with popular hooks like
useState
,useEffect
,useReducer
,useMemo
, anduseCallback
. - Context API: Learn how to avoid prop drilling by using the Context API.
- React Router: Understand routing basics, dynamic routes, and navigation.
- Practice Coding:
- Solve React-specific problems on LeetCode or HackerRank.
- Build personal projects to showcase your skills. Create apps like to-do lists or small dashboards to demonstrate real-world usage.
- Stay Updated:
- Follow React’s official documentation to learn about the latest features.
- Join React developer communities or forums to learn tips and tricks from experts.
If you’re new to React or want to deepen your skills, Codei5 Academy offers the best React JS courses in Coimbatore, available as live and online classes.
Interview Strategies
- Communicate Clearly:
- Explain your thought process while solving problems.
- Use simple and clear language instead of overly technical terms.
- Ask questions if you don’t understand the problem fully.
- Whiteboarding Practice:
- Break down big problems into smaller, manageable steps.
- Write neat, readable code with clear variable names.
- Think about edge cases and test your solutions.
- Be Ready for Behavioral Questions:
- Talk about your past projects and what you learned.
- Highlight teamwork, problem-solving, and adaptability in your answers.
- Prepare for common questions like, “Tell me about a challenge you faced and how you solved it.”
- Show Enthusiasm:
- Stay curious about the latest trends in React and web development.
- Ask thoughtful questions about the role and the company to show genuine interest.
Conclusion
By preparing consistently and practicing both technical and communication skills, you’ll increase your chances of success. For a solid foundation in React, consider Codei5 Academy’s React JS course, where you can learn from experts in both live and online formats. Good luck with your interview!