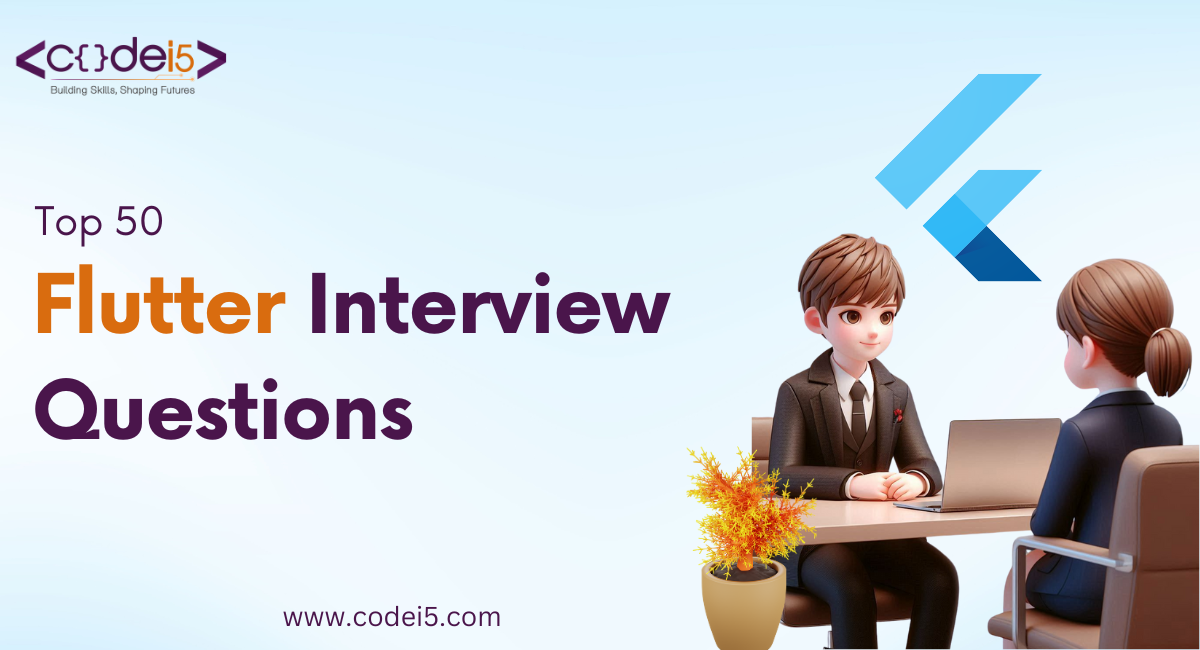
Are you ready to slay your Flutter interview? If you’re all about creating dope UIs and building awesome cross-platform apps, Flutter is where it’s at. It’s becoming the top choice for developers who want speed, flexibility, and a great user experience—all from one codebase. From fresh startups to big tech companies, everyone is hopping on the Flutter train.
But here’s the deal: how do you nail that interview? Don’t worry, we’ve got you covered! We’ve put together the top 50 Flutter interview questions that you’ll want to prep for, covering everything from the basics to the nitty-gritty details. You’ll see questions about Flutter’s architecture, state management, and how to boost app performance—plus some behavioral questions to see how you think on your feet.
Whether you’re new or leveling up, this guide will help you ace your interview and show you’re the Flutter superstar they want. Let’s get it!
Basic Flutter Interview Questions
1. What is Flutter?
Answer: Flutter is a UI toolkit created by Google that helps developers build high-performance, cross-platform mobile apps using a single codebase. It uses the Dart programming language and comes with a variety of widgets for creating beautiful and responsive user interfaces.
2. What are widgets in Flutter?
Answer: Widgets are the basic building blocks of Flutter apps. They represent UI elements like buttons, text fields, images, and more. Flutter’s widget-based structure makes it easy to create complex and customizable interfaces.
3. Explain the difference between Stateless and Stateful widgets.
Answer:
- Stateless widgets: These widgets don’t change over time. They are fixed and used for simple UI elements that don’t need to update.
- Stateful widgets: These widgets can change their state, allowing for dynamic updates and user interactions. They are used for more complex elements that need to respond to user input or changes in data.
4. How does Flutter differ from other mobile app frameworks like React Native?
Answer: Flutter differs from React Native in a few key ways:
- Rendering engine: Flutter has its own rendering engine (Skia), while React Native uses the native platform’s engine.
- Widget library: Flutter offers a more extensive and customizable widget library.
- Performance: Flutter often has better performance because it directly renders to the platform.
5. What is the role of Dart in Flutter?
Answer: Dart is the programming language used for building Flutter apps. It is modern and object-oriented, featuring asynchronous programming, strong typing, and hot reload capabilities.
6. What are keys in Flutter?
Answer: Keys are used to uniquely identify widgets within a widget tree. They help Flutter efficiently update and reuse widgets when the UI changes.
7. Explain the main() function in Flutter.
Answer: The main() function is the starting point of a Flutter app. It creates a runApp()
widget, which is the root widget of the app’s widget tree.
8. What is the purpose of pubspec.yaml?
Answer: The pubspec.yaml
file is a configuration file that outlines the dependencies and metadata for a Flutter project. It specifies the project’s name, version, and the external packages used.
9. What is a Scaffold widget in Flutter?
Answer: A Scaffold widget provides a basic structure for a Flutter app, including an app bar, drawer, floating action button, and a body where other widgets can be placed.
10. What are hot reload and hot restart in Flutter?
Answer:
- Hot reload: This feature lets developers see changes in their app immediately without restarting the whole app. It speeds up development and debugging.
- Hot restart: This feature restarts the app but keeps the current state. It’s helpful when you need to reload everything while retaining the current data.
Intermediate Flutter Interview Questions
1. Explain the Flutter build process.
Answer: The Flutter build process involves these steps:
- Compilation: Dart code is converted into machine code for the target platform (iOS, Android, or web).
- Widget tree creation: Flutter builds a tree of widgets based on your app’s code.
- Layout calculations: Flutter calculates where each widget will be placed on the screen.
- Painting: The widgets are drawn on the screen using the Skia graphics library.
2. What is a Future in Dart?
Answer: A Future in Dart represents a value that will be available at some point. It’s commonly used for asynchronous tasks, like fetching data from the internet or reading files.
3. What are Streams, and how do they work in Flutter?
Answer: A Stream in Dart is a sequence of data that can be sent over time. It’s useful for handling real-time updates, like data from a server. In Flutter, you can use StreamBuilder widgets to listen to streams and update the UI as new data comes in.
4. How do you manage state in Flutter?
Answer: Flutter provides several ways to manage state:
- Stateful widgets: For managing state within a single widget.
- Provider: A popular solution that uses dependency injection to share data across the app
- BLoC (Business Logic Component): A pattern that separates UI from business logic for cleaner code.
- Riverpod: A newer solution that offers a simpler and type-safe approach to state management.
5. What is Provider in Flutter, and how does it work?
Answer: Provider is a state management library that makes data accessible throughout your app without needing to pass it down through each widget. It uses dependency injection to provide data to other widgets easily.
6. Explain the difference between FutureBuilder and StreamBuilder.
Answer:
- FutureBuilder: Used to build UI based on a single future value.
- StreamBuilder: Used to build UI based on a stream of data, allowing for real-time updates.
7. How do you navigate between pages in Flutter?
Answer: Flutter uses the Navigator widget for navigation. You can use methods like pushNamed()
to go to a new page and pop()
to return to the previous one.
8. How do you create custom widgets in Flutter?
Answer: To create a custom widget, extend either StatelessWidget
or StatefulWidget
, and override the build()
method to define how the widget looks.
9. What is the purpose of the ‘Navigator’ widget?
Answer: The Navigator widget manages the navigation stack in your Flutter app. It keeps track of which page is currently displayed and allows you to switch between different pages.
10. How do you handle user input in Flutter?
Answer: Flutter provides various widgets for user input, such as:
- TextField: For entering text.
- Checkbox: For selecting true or false.
- RadioListTile: For choosing one option from several.
- DropdownButton: For selecting a value from a list.
You can use event handlers like onChanged
to capture user input and update the app’s state.
Advanced Flutter Interview Questions
1. How do you optimize the performance of a Flutter app?
Answer: To optimize your Flutter app’s performance, you can:
- Minimize widget rebuilds: Use
const
constructors for widgets that don’t change, and avoid unnecessary rebuilds. - Optimize layouts: Use efficient layout widgets and keep layouts simple.
- Reduce image size: Compress images and choose the right formats.
- Use async operations: Move heavy tasks to separate threads to keep the UI smooth.
- Profile your app: Use Flutter’s tools to find and fix performance issues.
2. What is the difference between runApp()
and build()
in Flutter?
Answer:
runApp()
: This function starts the Flutter app and creates the main widget tree.build()
: This method is called to render the widget’s UI. You override it inStatelessWidget
andStatefulWidget
to define how the widget looks.
3. How does Flutter handle gestures?
Answer: Flutter has gesture detectors that recognize different actions like taps, swipes, and drags. You can combine these detectors to create more complex interactions.
4. What is the Flutter BLoC pattern?
Answer: The BLoC (Business Logic Component) pattern is a way to separate the UI from the business logic in Flutter. It makes the code easier to maintain and test by keeping concerns separate.
5. Explain the concept of ‘inherited widget’ in Flutter.
Answer: Inherited widgets let you share data down the widget tree without passing it to each widget as an argument. This is useful for data that many widgets need access to.
6. What is the role of the ‘BuildContext’?
Answer: The BuildContext provides information about a widget’s position in the widget tree. It helps access themes, localization, and other important data.
7. How do you implement animations in Flutter?
Answer: Flutter offers a robust animation system that lets you create:
- Tween animations: Animate values between two points.
- Curve animations: Control how animations speed up or slow down.
- Physics-based animations: Create realistic movements using physics.
8. What are Slivers in Flutter?
Answer: Slivers are special widgets for creating custom scrollable layouts. They help build flexible UI components like app bars, footers, and drawers.
9. Explain platform channels in Flutter.
Answer: Platform channels let Flutter communicate with native code on iOS or Android. They enable access to features that aren’t available in Flutter.
10. How do you debug a Flutter application?
Answer: Flutter offers several debugging tools:
- Debugger: Step through code, set breakpoints, and check variables.
- Hot reload: See changes instantly without restarting the app.
- Performance profiling: Measure performance and find issues.
- Logcat: View logs from the device.
- Flutter Inspector: Visualize the widget tree and inspect widget properties.
Behavioral and Problem-Solving Questions
1. Describe a challenging Flutter project you worked on. How did you overcome the challenges?
Answer: Talk about a specific project where you faced a major challenge. Explain the steps you took to overcome it, like doing research, collaborating with your team, or looking for help online. Focus on your problem-solving skills and how you remained determined.
2. How do you stay updated with the latest trends and updates in Flutter?
Answer: Share how you keep yourself informed, such as reading Flutter blogs, subscribing to newsletters, attending conferences, or working on open-source projects. Emphasize your commitment to continuous learning and adapting to new technologies.
3. Can you explain a time when you optimized the performance of a Flutter app?
Answer: Provide an example where you found a performance issue and improved the app’s speed or responsiveness. Describe the techniques you used, like reducing widget rebuilds or optimizing layouts, and how these changes enhanced the user experience.
4. What are the most common mistakes developers make in Flutter, and how do you avoid them?
Answer: Discuss common issues like performance problems, memory leaks, or poor state management. Explain how you avoid these mistakes by following best practices, using code analysis tools, and conducting regular code reviews.
5. How do you approach debugging and fixing bugs in a Flutter app?
Answer: Describe your step-by-step approach to debugging, which includes using debugging tools, checking logs, and isolating the root cause of the problem. Talk about how you break down complex issues into smaller parts and stay persistent in finding solutions.
6. Have you contributed to any open-source Flutter projects?
Answer: If you have, describe your experiences and the contributions you made. Highlight the benefits of open-source work, like learning from others, helping the community, and improving your skills.
7. How do you prioritize feature development in a Flutter app?
Answer: Explain how you prioritize features by considering user needs, business goals, and technical feasibility. Discuss how you balance the need for quick development with the importance of maintaining quality and ensuring long-term maintainability.
8. Can you describe a time when you disagreed with a team member about an approach to solving a Flutter-related issue?
Answer: Share a situation where you disagreed with a team member. Explain how you addressed the disagreement, communicated openly, and worked towards a solution that benefited the project. Highlight your ability to collaborate and find common ground.
9. What’s your experience working with Firebase in Flutter apps?
Answer: Discuss your experience with Firebase, mentioning specific features you’ve used, like authentication or databases. Share any challenges you faced and how you overcame them.
10. How do you handle app performance when dealing with large amounts of data in Flutter?
Answer: Explain your strategies for improving app performance with large datasets, such as using pagination, caching, or efficient data structures. Discuss your experience with performance testing and optimization tools.
Scenario-Based Flutter Questions
1. How would you implement lazy loading in Flutter?
Answer: I would use the ListView.builder
widget to create a list that loads items as the user scrolls. I’d fetch data in batches from a network or database and add it to the list as needed. This helps improve performance by avoiding loading all data at once.
2. How would you integrate REST APIs into a Flutter app?
Answer: I would use the http
package to make requests to the REST API. I’d handle different response codes and errors properly. For authentication, I could use methods like OAuth or API keys. To manage the data, I might use a state management solution like Provider or BLoC.
3. What would you do if an animation wasn’t working as expected in Flutter?
Answer: First, I would check the animation code for issues, such as incorrect timing or settings. I would use debugging tools to visualize the animation and go through the code step-by-step. If problems continued, I would simplify the animation or look for troubleshooting tips online.
4. How would you handle network connectivity issues in a Flutter app?
Answer: I would use the Connectivity plugin to monitor the network status and show messages or loading indicators as needed. I’d implement retry logic for failed network requests and add offline capabilities if required.
5. How do you deal with app crashes or unexpected behavior in Flutter?
Answer: I would use Flutter’s error handling features, like try-catch blocks and ErrorWidgets. I’d also enable crash reporting tools to gather information about errors and spot patterns. For debugging, I would use the Flutter debugger and log messages.
6. What steps would you take to internationalize a Flutter app?
Answer: I would use Flutter’s MaterialLocalizations and Globalizations classes to manage different languages. I would create specific files for strings, images, and resources for each language. Additionally, I’d handle date and number formatting based on different cultures.
7. How would you implement dark mode in a Flutter application?
Answer: I would use the ThemeData
class to define themes for both light and dark modes. I’d use the Theme widget to apply the correct theme based on the user’s preferences or system settings, ensuring that the app’s UI elements are easy to see in both modes.
8. How would you secure a Flutter app?
Answer: I would follow security best practices like:
- Using HTTPS for secure network communication.
- Validating user input to avoid attacks.
- Storing sensitive data securely with encryption.
- Implementing strong authentication and authorization.
- Regularly updating dependencies to fix security issues.
9. How would you use platform-specific features in a Flutter app?
Answer: I would use platform channels to communicate with native code and access features like the camera or GPS. I’d carefully consider the balance between using cross-platform features and needing specific native functionality.
10. How do you handle version control and code review when working on a Flutter team?
Answer: I would use a version control system like Git to track changes and work with my team. I’d follow set strategies for branching and merging. I would also take part in code reviews to maintain code quality and consistency.
Conclusion
Congratulations on finishing this detailed guide to Flutter interview questions! By learning the topics in this blog, you’ve made a big step toward becoming a skilled Flutter developer.
Remember, a successful Flutter interview combines technical knowledge with good communication skills. It’s important to know Flutter’s core concepts, but it’s also crucial to show your problem-solving abilities, teamwork, and enthusiasm for mobile app development.
Here are some tips to help you prepare for your interviews:
- Practice coding: Regularly code Flutter applications to strengthen your understanding of the framework and improve your problem-solving skills.
- Build personal projects: Create your own Flutter projects to showcase your skills and gain hands-on experience.
- Stay updated: Follow blogs, attend conferences, and contribute to open-source projects to keep up with the latest trends in Flutter.
- Prepare for behavioral questions: Practice answering behavioral questions to show your soft skills and how they connect to Flutter development.
If you want to ace your Flutter interviews and are a beginner starting your career in Flutter, consider visiting Codei5 Academy, which offers the best Flutter courses with both live and online classes.
By following these tips and using the information from this blog, you’ll be ready to confidently handle any Flutter interview question. Good luck!